Here you can read all about my custom Unity third person character controller. Currently, this is only 1 script and it has basic functionalities like walking, jumping, sprinting and camera movement. I made this in C# and I will continue to develop this script by adding more functionalities like animations, base code for picking up items and combat system.
Movement Functionality
For the movement mechanic I used the Rigidbody component, because that would give the character the ability to interact with physics objects. You can see the actual code on my Github page here.
When making this script, I had the option to choose between using the Character Controller component or using the Rigidbody component. Both componenets have pros and cons. The Character Controller is easier to use for movement and already has a lot of built in funcionality, but it can’t interact with physics objects. Using the Rigidbody for the movement has more advantage if the player/character has to interact with physics objects, but it doesn’t have a lot of built in functionality that is used for movement.
I chose to go with the Rigidbody component, because I wan’t to build my own custom character controller and using the character controller would be cheating in my eyes.
How the actual movement is implemented is quite simple. I based my movement on vectors. I begin with a normalized 3d vector which stores the input of the keyboard (so if I press the A or the left key it will be [-1, 0, 0] and if I press the W or up key it will be [0, 0, 1]). I also do some calculations to make the character rotate towards the direction it is moving, so it won’t jump to that direction but rotate towards that direction. When all that is calculated I update the rigidbody position with a normalized velocity * movement speed * delta time and if the player is sprinting the movement speed will be multiplied by 1.5 by default.
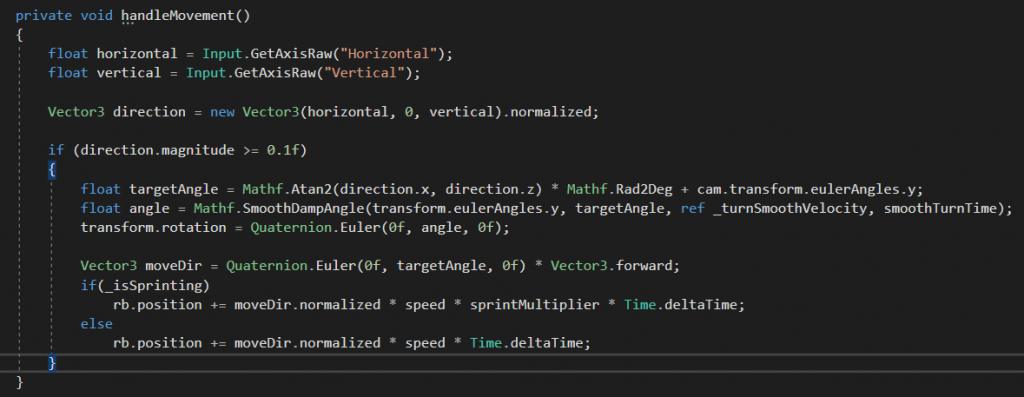
Camera Functionality
For the camera controls I used the Unity cinemachine, it makes it really easy to control the camera . I used the cinemachine camera, because my focus was on making the movement for the player and not the camera. I am planning on making a custom script for the camera controls for a third person character.
There isn’t a whole lot to say about the functionality of the camera movement, since the cinemachine takes care of all the functionality it needs.
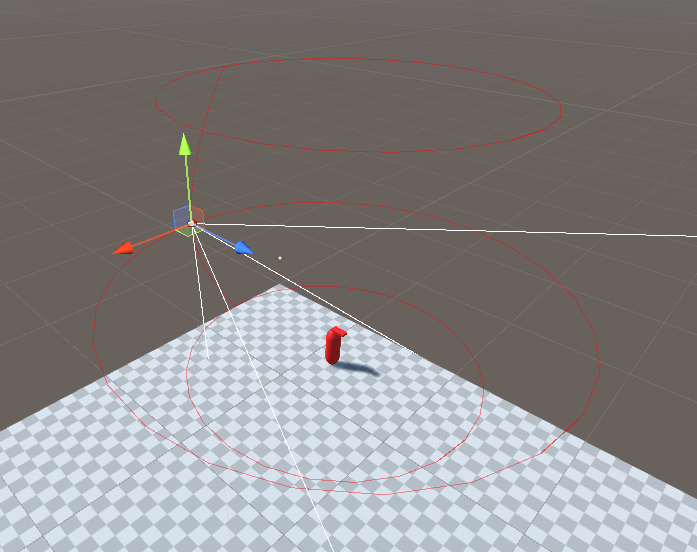
Here you can find the Github repo: https://github.com/JdeHaan2001/CustomUnityCharacterController