Well hello there and thank you for looking at my small arcade game.
This game is a simple scene of an arcade like game. It was made in C++ with SFML and all the art was free art from the internet. I am still developing and trying to better my C++ skills, so what I can show is still quite limited.
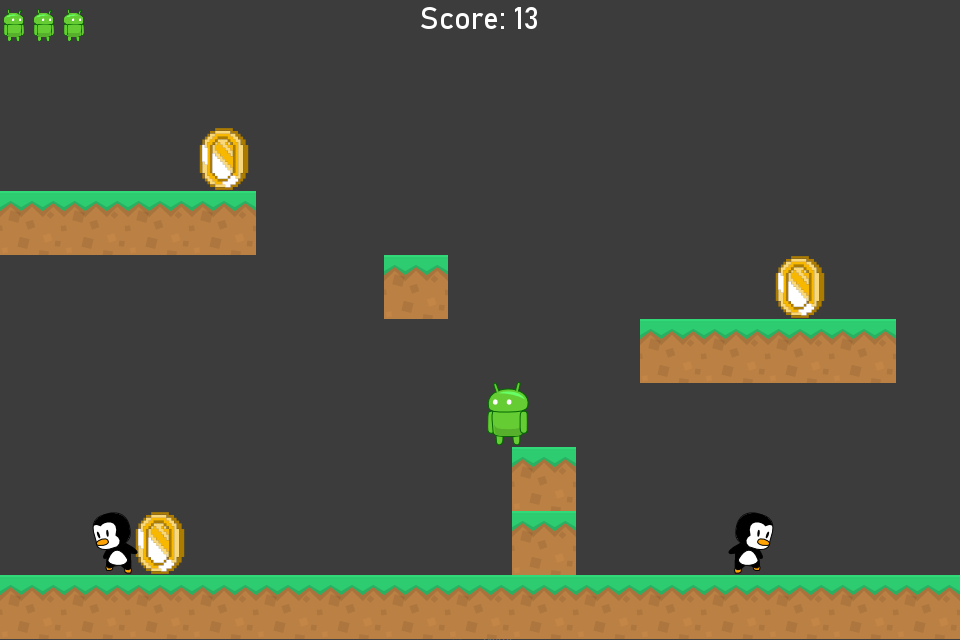
Game Mechanics
It is a simple game where the player (android character) has to pickup all the coins and not get hit by the evil penguins. The player’s score is based on how many coins he has picked up and how long he’s alive, because every second the player is alive 1 point get added to the score.
Right now the penguings have a very simple “wandering” behaviour. They’re always walking around and change direction once they hit something like a wall or the edge of the screen. If an enemy hits a player the player goes back to the starting position and loses 1 live. If the player loses all his lives than the game will be over.
How I made it
As I said before, I made this project in C++ with SFML. I didn’t use a fancy game engine, because I wanted to gain more experience in C++ programming so I didn’t want to use a game engine.
When I started working on the game I started by making 3 seperate projects. I made a project for the player, enemy and for a map loading system. I did this so that I could focus on 1 thing at the time and not have to worry about adding more “advanced” functions (e.g. collision between player and the level) before I could start making the more basic functions (e.g. movement).
Before I started this project I already had some classes that I re-used for this project. For example a GameObject base class which contains some basic functions that every object that needs to be shown on screen needs to have (e.g. an update and a render function) or a Scene and Scene Handler class, so that I could make a class for each scene and that it would be easy to switch between scenes.
Player
The player’s functionality is quite basic. The player can walk around, jump and interact with enemies and coins. For the player’s movement I used vectors, so the player has a velocity vector that gets multiplied with speed.
The collision with the tile map is based on which tile there is in the map, currently there is a collision check with every tile in the map. Which is fine for now, performance wise, because it is a small map. But if there was a bigger tile map I would only do collision checks on tiles that the player was able to collide with.
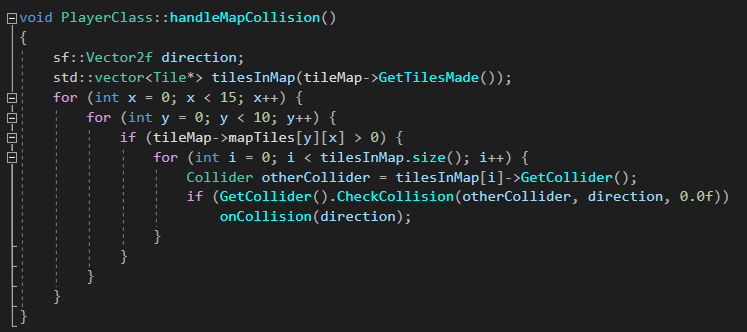
Enemy
For the enemy I didn’t need a very complex behaviour. I just needed it to walk in 2 directions (left & right) and have it affected by gravity, so that if it falls from a platform it actually falls down.
I did the movement the same way as for the player. Only I had an integer variable that was either 1 or -1 to give the direction of the enemy. So -1 would be left and 1 would be right. So the only thing I had to change for the player to change direction was that integer variable.
In the enemy project I didn’t have to think about collision with the map yet, I only had it change direction when it collided with the edge of the screen. When I made the game itself I did the collision with the tile map the same way as I did with the player.
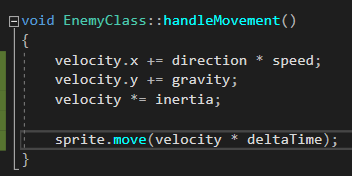
Map Loading
To make the tile map, I have a for loop that loops through a 2d array which represents the tilemap. In that for loop I can decide which tile is used for which number in the array. This gives me a lot of opportunity to customize the map.
Right now I set the 2d array in the header file, but I want to change it to a system where I can read the array from a .txt or .json file so that it’s even easier to implement new tile maps into the game.
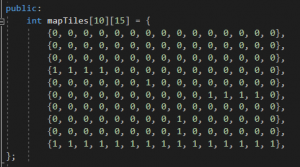
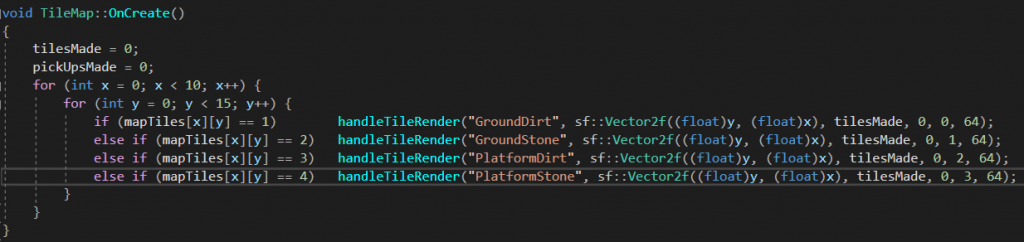
Here you can find the repo for the code: https://github.com/JdeHaan2001/PersonalPortfolio3